Create pagination for Vue project using Laravel backend
Hello friends, in this article I am going to teach you how to create pagination for you Vue app using Laravel backend. You may think this process might be easy, ya it is easy if you are developing both Vue and Laravel project together. But if we using Laravel backend as API and if Vue and Laravel are separate projects, this may be little bit hard. However I am going to show the easiest way to integrate pagination for your Vue project. So before we start we have to add a dependency to the Vue project. Now locate to your Vue project directory and open via CMD and add following dependency.
If you are using NPM
npm install laravel-vue-pagination
If you are using Yarn
yarn add laravel-vue-pagination
Ok. Now lets go to the Laravel backend first. Follow the below steps.
First of all create the route for your endpoint in web.php
If you are using Laravel pure framework
Route::get('/fetchusers', 'UserController@fetchAllUsers');
Or if you are using Laravel Lumen framework
$router->get('/fetchusers', 'UserController@fetchAllUsers');
If you have any issue on routing go Laravel official document link given below
https://laravel.com/docs/7.x/controllers
Ok. Now we have to write the code in our controller to send the users data as a Json API object with pagination. Go to UserController
public function fetchAllUsers()
{
$users = User::paginate(3);
return response()->json($users, 200);
}
So the above function will return the user data as a json object with limiting 3 user data per page.
Now lets move to Vue frontend
In the frontend we are using axios to retrieve the API from Laravel backend. So now create a page or component.
In the template section use below code
<template>
<div>
<ul v-for="(user,index) in userData.data" :key="index">
<li>{{user.first_name}}</li>
</ul>
<pagination :data="userData" @pagination-change-page="getPaginatedData"></pagination>
</div>
</template>
In the script section
<script>
import Vue from 'vue'
Vue.component('pagination', require('laravel-vue-pagination'));
export default {
name: 'PaginationTest',
data () {
return {
userData: [],
}
},
created(){
/* NOTE- If this.$axios not worked try just axios. */
let response = this.$axios.get("fetchusers").then(response => {
console.log(response.data)
this.userData = response.data;
})
.catch(error => {
console.log('error messsage: ', error.message)
})
},
methods:{
getPaginatedData(page = 0){
/* NOTE- If this.$axios not worked try just axios. */
let response = this.$axios.get("fetchusers?page="+page).then(response => {
this.userData = response.data;
})
.catch(error => {
console.log('error messsage: ', error.message)
})
}
}
}
</script>
NOTE – You might be wondered how and why we are using “fetchusers?page=” endpoint without defining the route in backend web.php. Its because laravel will automatically create it route for the pagination. I have used the console.log at first time when we fetching the data. If you see at the response, it is different from other response we getting from backend. In that case only when we using for loop we have to use userData.data without using just userData. you can see the example response given below
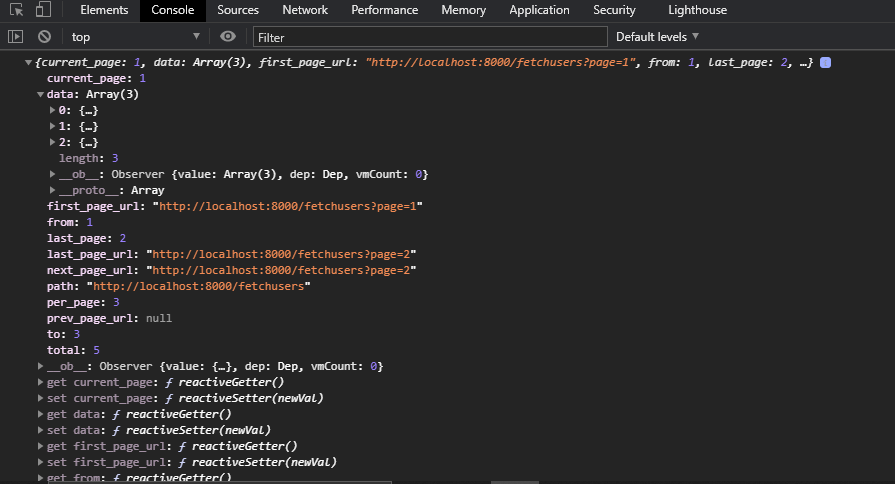
And finally, if your pagination look like a horizontal list use following style to make it vertical and remove the dots
<style>
.pagination{
list-style: none;
display: flex;
}
.pagination > li {
padding-right : 10px;
}
</style>
Thats it! if you have any questions please comment below. Or if you want more articles, go to below link.